Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 3224 | Accepted: 2354 |
Description
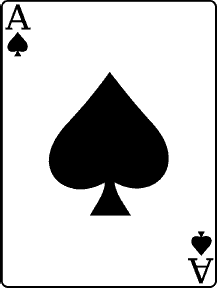
The magician shuffles a small pack of cards, holds it face down and performs the following procedure:
- The top card is moved to the bottom of the pack. The new top card is dealt face up onto the table. It is the Ace of Spades.
- Two cards are moved one at a time from the top to the bottom. The next card is dealt face up onto the table. It is the Two of Spades.
- Three cards are moved one at a time…
- This goes on until the nth and last card turns out to be the n of Spades.
This impressive trick works if the magician knows how to arrange the cards beforehand (and knows how to give a false shuffle). Your program has to determine the initial order of the cards for a given number of cards, 1 ≤ n ≤ 13.
Input
On the first line of the input is a single positive integer, telling the number of test cases to follow. Each case consists of one line containing the integer n.
Output
For each test case, output a line with the correct permutation of the values 1 to n, space separated. The first number showing the top card of the pack, etc…
Sample Input
245
Sample Output
2 1 4 33 1 4 5 2
Source
题意:给你n张牌,让你变一个魔术:第1次把上面的1张牌放到底部,然后最上面的牌就是1,然后拿走1。第2次把上面的2张牌依次放到底部,然后最上面的牌就是2,然后拿走2....重复这个过程,直到所有的牌都被拿走。问一开始的牌应该从上到下怎么放,才能完成这个魔术。
算法:逆向思维,从后向前模拟。不要“下盲棋”,拿几张牌试试就明白了。。。
#include#include #include #include using namespace std;int n;queue q;void output(){ // 递归输出q里面的内容 int tmp=q.front(); q.pop(); while(!q.empty()) output(); printf("%d ",tmp);}int main(){ //freopen("input.txt","r",stdin); int t; scanf("%d",&t); while(t--){ scanf("%d",&n); int cnt; while(n>0){ q.push(n); cnt=n; n--; while(cnt--){ // 由后向前翻cnt次牌 int tmp=q.front(); q.pop(); q.push(tmp); } } output(); printf("\n"); } return 0;}